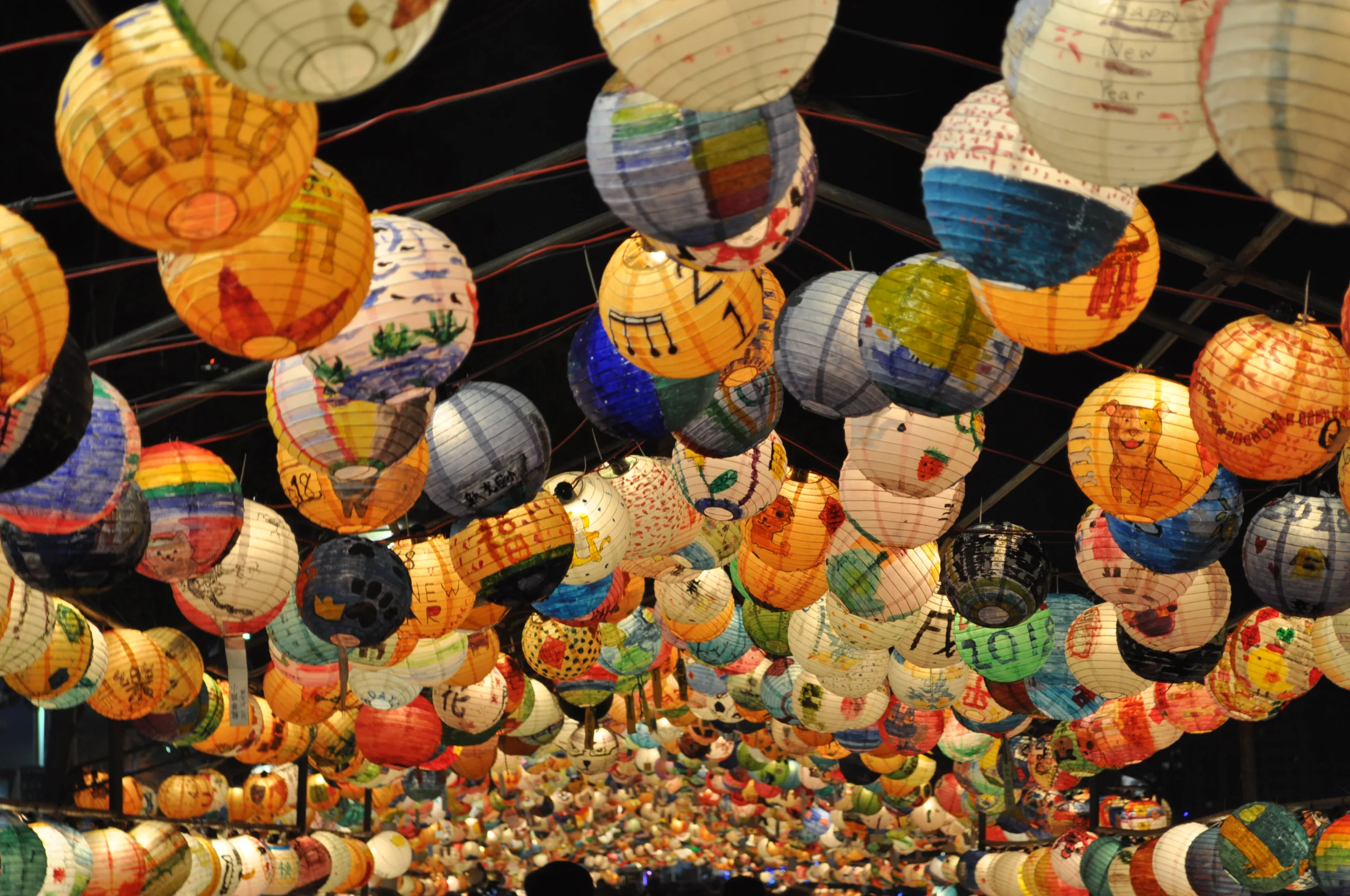
Bridge
Bridge Concept
Bridge pattern is a structural design pattern. The concept involves further subdividing the features or behaviors of an existing object, aiming to achieve the separation of the object’s behavior.
In common scenarios, objects inherited through layers of inheritance tend to become increasingly complex.
The advantage of this pattern is the ability to replace the originally inherited behavior with a bridged approach, where shared interface methods are invoked within the object.
The concrete methods are then provided externally.
Bridge Example
//**************
//* author: cian
//* 20231024
//**************
interface IConnector
{
void Connect();
void Disconnect();
void WriteCmd(byte[] cmd);
}
class Ethernet : IConnector { /*Implement*/ }
class SerialPort : IConnector { /*Implement*/ }
interface IPacket
{
byte[] GetOpenCmd();
byte[] GetCloseCmd();
}
class EthernetPacket : IPacket { /*Implement*/ }
class SerialPacket : IPacket { /*Implement*/ }
class Controller
{
private IConnector Connector;
public Controller(IConnector connector)
{
Connector = connector;
}
public void Connect() { Connector.Connect(); }
public void Disconnect() { Connector.Disconnect(); }
public void OpenControl(IPacket packet)
{
Connector.WriteCmd(packet.GetOpenCmd());
}
public void CloseControl(IPacket packet)
{
Connector.WriteCmd(packet.GetCloseCmd());
}
}
Conclusion
Upon reflection, I find the concepts of this pattern and Abstract Factory quite similar. I decided to experiment by creating a similar example, with the distinction that Abstract Factory is responsible for creation, while this pattern focuses on abstracting behaviors within the original object.
In my case, I abstracted the packaging behavior, simplifying the abstract class ControllerBase
, which was originally inherited from the Abstract Factory, into a regular class. I’m uncertain whether my understanding is entirely accurate and would appreciate confirmation.
Leave a Reply