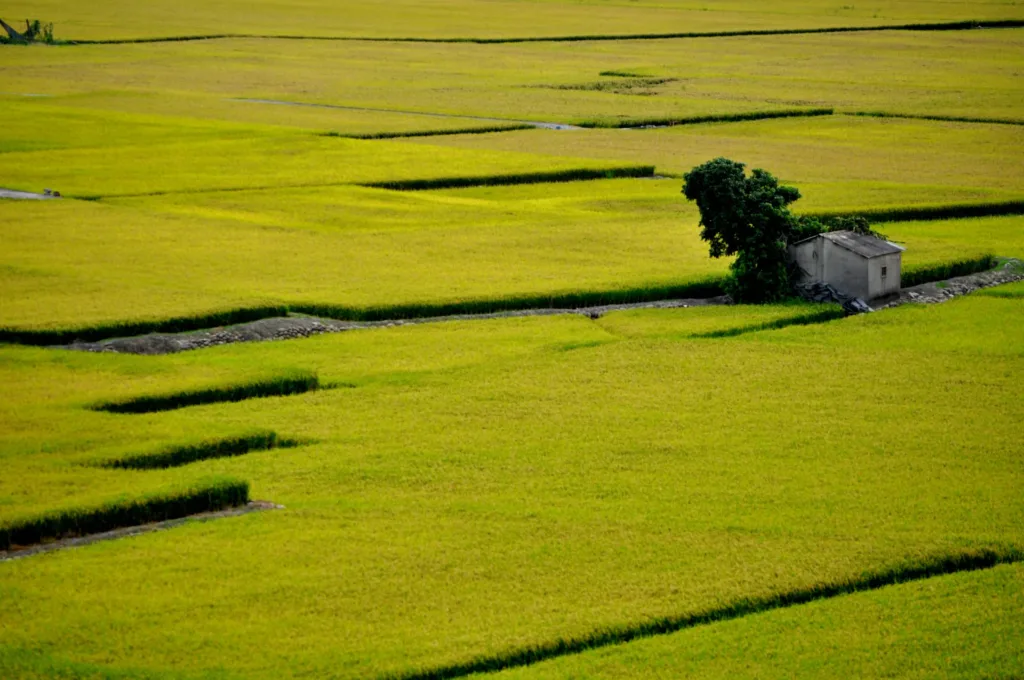
Prototype
Prototype Concept
The Prototype pattern is a creational design pattern that aims to reduce the cost of creating objects by copying them.
In C#, it is relatively easy to achieve object cloning by implementing the ICloneable interface and the Clone() method.
Shallow Copy & Deep Copy
Furthermore, object cloning can be categorized into two types: Shallow Copy & Deep Copy.
The key difference lies in the Clone() process. In Shallow Copy, for reference types, only the object references are copied. In Deep Copy, the entire object is duplicated.
For value types, they are always copied regardless of the cloning type.
Therefore, if an object contains instances of other classes, Deep Copy should be used; whereas, if it consists only of value types, Shallow Copy may be sufficient.
Prototype Example
Reference types need to be copied separately; otherwise, the members within the copied object will still reference the same objects as the original.
//**************
//* author: cian
//* 20231022
//**************
class MyClass : ICloneable
{
private int A = 100;
private string B = "my text";
private SubClass C = new SubClass();
public object Clone() //Deep Copy
{
MyClass obj = (MyClass) this.MemberwiseClone();
obj.C = (SubClass) this.C.Clone();
return other;
}
}
class SubClass : ICloneable
{
private int A = 100;
private string B = "subClass";
public object Clone() //Shallow Copy
{
return this.MemberwiseClone();
}
}
Conclusion
In C#, there is a universal method for achieving complete object cloning through deep copy, eliminating the need to consider internal types or manually copy each member.
However, for the purpose of distinguishing between the two types of copying, here emphasizes a more approach to highlight the differences.
Leave a Reply