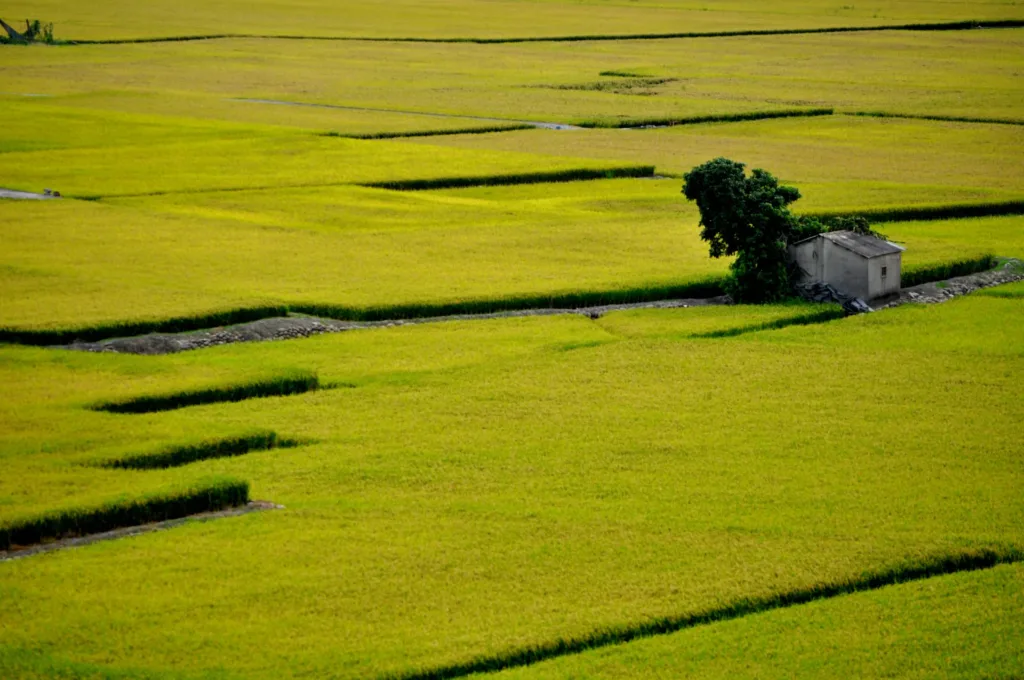
Abstract factory
Concept
The Abstract Factory is a creational pattern that extends the concept of the Factory Method. It abstracts the factory layer, allowing the creation of objects from a common factory for a specific theme, rather than generating more factories.
In the previous example of the Factory Method, two common industrial communication methods (Ethernet and SerialPort) were used. This time, we further extend the concept by implementing the control functions of the controller.
Assuming the controller has two methods: turn on and turn off, and the packet for different communication are not interchangeable. Ethernet corresponds to one type of packet, and SerialPort corresponds to another type of packet.
Additionally, placing the packet code inside the communication methods violates the principle. In such cases, the pattern aligns with a thematic structure, providing a solution.
Abstract Factory Example
//**************
//* author: cian
//* 20231023
//**************
enum ControllerEnum
{
TYPE_A,
TYPE_B,
}
interface IConnector
{
void Connect();
void Disconnect();
void WriteCmd(byte[] cmd);
}
class Ethernet : IConnector { /*Implement*/ }
class SerialPort : IConnector { /*Implement*/ }
interface IPacket
{
byte[] GetOpenCmd();
byte[] GetCloseCmd();
}
class EthernetPacket : IPacket { /*Implement*/ }
class SerialPacket : IPacket { /*Implement*/ }
abstract class ControllerBase
{
public abstract IConnector Connector { get; protected set; }
public abstract IPacket Packet { get; protected set; }
public virtual void Connect() { Connector.Connect(); }
public virtual void Disconnect() { Connector.Disconnect(); }
public virtual void OpenControl()
{
Connector.WriteCmd(Packet.GetOpenCmd());
}
public virtual void CloseControl()
{
Connector.WriteCmd(Packet.GetCloseCmd());
}
}
class TypeA : ControllerBase
{
public override IConnector Connector
{ get; protected set; } = new Ethernet();
public override IPacket Packet
{ get; protected set; } = new EthernetPacket();
}
class TypeB : ControllerBase
{
public override IConnector Connector
{ get; protected set; } = new SerialPort();
public override IPacket Packet
{ get; protected set; } = new SerialPacket();
}
static class ControllerFactory
{
public static ControllerBase GetController(ControllerEnum controller)
{
switch (controller)
{
case ControllerEnum.TYPE_A:
return new TypeA();
case ControllerEnum.TYPE_B:
return new TypeB();
default:
throw new NotImplementedException();
}
}
}
Conclusion
If in the future, there is a need to add a third type of controller, it would only be necessary to confirm the communication method and implement the packet format.
The Abstract Factory Pattern allows for the creation of multi-layered software architectures. By adhering to the actual program structure, one can write elegant code and implement beautiful software solutions.
Leave a Reply